
In Javascript an array is an ordered list made up of values. Each value in the array is called an element, and elements are determined by an index. New programmers often battle with learning data structures and arrays because they’re tricky concepts to grasp.
To help remind yourself of things you've learned, never hesitate to incorporate cheat sheets into your programming journey. They're a great tool for picking up new methods and refreshing yourself on tricks you already know.
If you're brand new to coding and looking to dip your toes into computer science before you dive into arrays, the CS50 Data Structure Lecture should be at the top of your watch list.
CS50 is an introductory Computer Science course at Harvard university taught by David J. Malan. The video offers a foundational premise of the intellectual enterprises of computer science and the art of programming, with energy, depth, and clarity.
Javascript Array Cheat Sheets
When learning Javascript, it can be easy to forget the methods and functions that are at the heart of arrays and data structures. Remember, you don't need to know everything at once. Using a cheat sheet is completely normal at all levels of programming.
How does the saying go? Cheaters never win, winners never cheat? Not applicable here. We've put together a list of Javascript array cheat sheets to help you out.
1. Javascript Arrays | Javascript Tutorial For Beginners
You caught me. This one isn’t a cheat sheet. It’s a video tutorial from Dev Ed. Before you start collecting cheat sheets, it’s important to understand what arrays are, when they should be used, and how to create them – making this video a great starting point. Javascript Arrays is part of a larger series by Dev Ed, Javascript Tutorial for Beginners.
2. JavaScript Arrays Reference Cheat Sheet and Breakdown — Mutating Methods
Next up are JS mutable methods. Mutable methods mutate the original object once implemented. Hint: If you find yourself getting mutable and immutable mixed up, just remember that mutable = mutates.
To help keep these straight, Aaron Yu mapped out 9 mutating methods for arrays and separated them into four categories:
- Methods that return a modified array in place
- Methods that return a value
- Methods that return the new length of the array
Throughout his guide, Yu gives supportive examples of each method mentioned. Below, you’ll find Yu’s guide to non-mutating methods.
3. JavaScript Arrays Reference Cheat Sheet and Breakdown — Non-Mutating Methods
A non-mutating (or immutable) method is a method that does not change or alter the object after using the said method. This guide will help you identify methods that accept arguments and callback functions and which methods that will not accept arguments.
In part three of his series, Aaron Yu compiled a list of non-mutating methods for Javascript arrays. In total, you’ll get your hands on 25 useful immutable methods to have at-the-ready next time you need a refresher.
4. JavaScript Array Functions Cheat Sheet
This zero-fluff cheat sheet offers 31 Javascript array functions for you to reference while you work. Notably, this reference sheet gives each function a usability grade for different browsers through MDN and caniuse.com. For example:
flatMap() has a lower browser usability score than join().
A link to an in-depth browser usability report is provided for each function by clicks on the score icon.
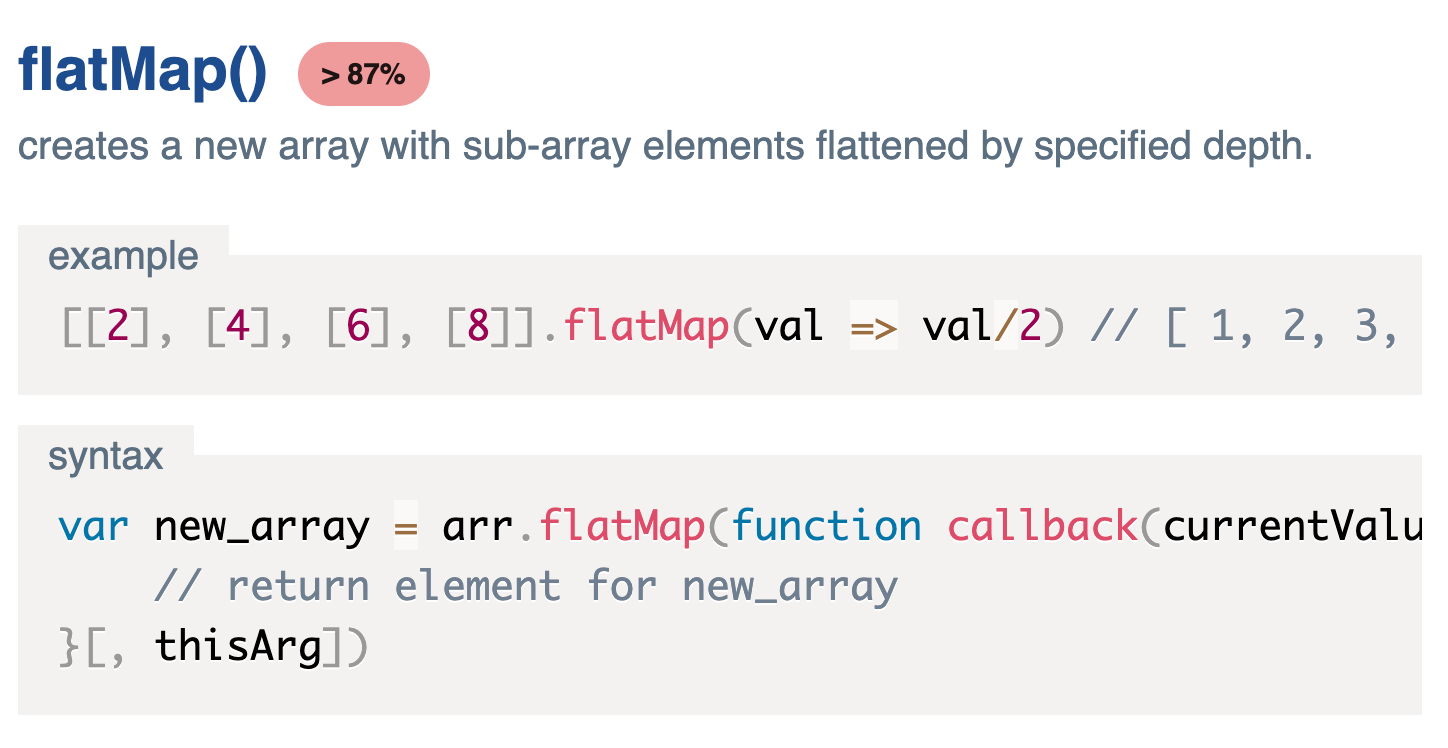

5. r/learnjavascript: Javascript array functions cheat sheet (as asked)

Since a picture’s worth a thousand words, this Javascript array cheat sheet graphic speaks for itself. Frame and place it on your desk for easy access. Additionally, r/learnjavascript is a great subreddit with a community of people learning Javascript alongside you. Community rule #1 states: “n00bs are welcome here. Negativity is not.”
6. How to Use the slice() and splice() JavaScript Array Methods
slice() and splice() may sound alike, but they’re actually two different methods used when working with Javascript arrays. Freecodecamp created a quick cheat sheet to provide context around the differences between slice() and splice().
Hint: slice() is immutable while splice() is mutable.
7. The JavaScript Array Handbook
Tapas Adhikary admits there are countless articles on JavaScript Arrays. However, in his time mentoring, he’s noticed that beginners need a thorough guide with lots of examples that fully explains the subject from beginning to end. That’s why he created The JavaScript Array Handbook. It’s packed with guides, examples, and explainers to help you learn array basics, methods, and more.